728x90
반응형
App Switcher - 앱 화면 가리기
iOS에서는 홈바 스와이프 또는 홈 버튼 두번을 클릭해 App Switcher를 사용할 수 있다.
이때 카카오톡, 은행앱 화면이 Blur나 다른 화면으로 가려지는데 이 기능을 어떻게 만들 수 있는지 알아보기로 한다.
App Switcher(앱 전환하기)는 뭘까?
- App Switcher 사용법 https://support.apple.com/ko-kr/HT202070!
- App Switcher는 다른 앱으로 빠르게 전환할 수 있도록 하는 기능이다. 아마 대부분 이름은 몰라도 아래 사진을 보면 어떤 기능인지 알고 있을 것이다.
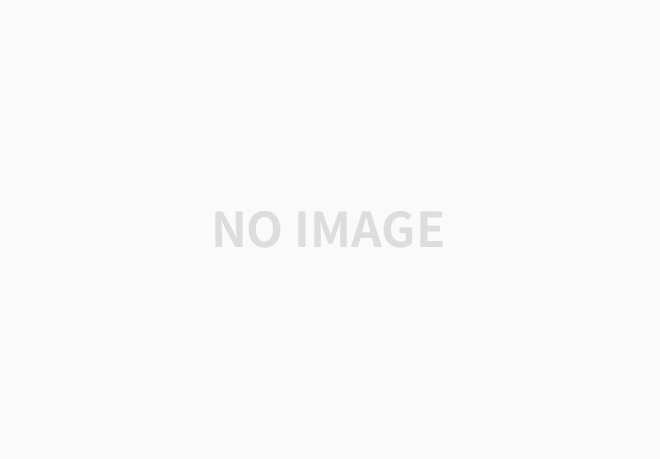
어떻게 하면 화면을 가릴 수 있을까?
- App switcher 상태가 감지되면 화면을 바꾼다. 앱이 실행되면 다시 화면을 바꾼다.
App Switcher 상태 감지
- blur나 이미지 처리를 하기 위해서는 현재 상태가 app switcher 중인지 체크를 해야한다.
SceneDelegate
를 사용하는 프로젝트를 기준으로 앱이 실행될 때- app switcher 모드 진입 시
sceneWillResignActive
가 호출된다. (AppDelegate
를 사용 중이라면applicationWillResignActive
) - 앱을 실행하면
sceneDidBecomeActive
가 호출된다.
- app switcher 모드 진입 시
- 그럼
sceneWillResignActive
에서 앱 화면이 안보이도록 해주고sceneDidBecomeActive
에서는 원래 화면이 보이도록 처리를 해주면 되겠다.
sceneWillResignActive
란?
- https://developer.apple.com/documentation/uikit/uiscenedelegate/3197919-scenewillresignactive
- willResignActive는 시스템 경고, 전화 수신 등 일시적인 중단을 위해 호출되며 또한 백그라운드 진입 전 호출되는 메서드이다.
- 공식문서에서 게임 앱은 게임을 중지시키고 이 모드가 실행 중일 때는 최소한의 작업을 하라고 명시되어 있다. 또 실행 중인 작업이 유실되지 않도록 데이터를 저장하는 과정도 필요하다고 되어있다.
sceneDidBecomeActive
란?
- https://developer.apple.com/documentation/uikit/uiscenedelegate/3197915-scenedidbecomeactive
- didBecomeActive는 scene이 활성화되고 사용자 이벤트에 응답하고 있음을 알리는 메서드이다.
- 이 URL에서 foreground 상태로 왔을 때 해야하는 일들을 알려주고 있다. https://developer.apple.com/documentation/uikit/app_and_environment/scenes/preparing_your_ui_to_run_in_the_foreground
이제 기능을 구현해보자!
이미지로 바꿔주기
sceneWillResignActive
에서 이미지로 덮어 씌우고sceneDidBecomeActive
에서 이미지를 없앤다
import UIKit
class SceneDelegate: UIResponder, UIWindowSceneDelegate {
var window: UIWindow?
// TODO: 1. 이미지뷰 생성
var imageView: UIImageView?
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
guard let _ = (scene as? UIWindowScene) else { return }
}
func sceneDidBecomeActive(_ scene: UIScene) {
// TODO: 3. 앱이 다시 활성화 상태가 되면 이미지뷰를 superview (window)에서 제거한다
if let imageView = imageView {
imageView.removeFromSuperview()
}
}
func sceneWillResignActive(_ scene: UIScene) {
// TODO: 2. will resign active 상태가 호출되면 imageview를 window 크기로 잡아주고 window에 추가한다
guard let window = window else {
return
}
imageView = UIImageView(frame: window.frame)
imageView?.image = UIImage(named: "image")
window.addSubview(imageView!)
}
}
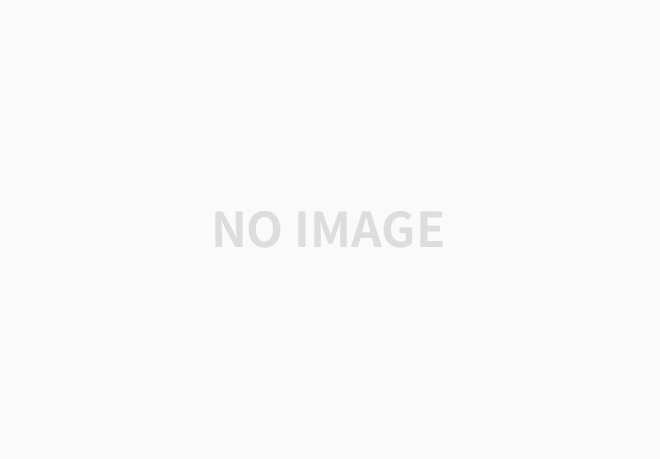
Blur로 바꿔주기
UIVisualEffectView
를 이용해 Blur 효과를 만들어줄 수 있다.- https://developer.apple.com/documentation/uikit/uivisualeffectview
UIVisualEffectView
는 원하는 효과에 따라 뷰 뒤에 레이어된 콘텐츠나 시각 효과 뷰에 추가된 콘텐츠에 영향을 줄 수 있다.- 주의점은 알파값을 1 미만으로 하면 안된다. 효과가 올바르지않게 보이거나 표시되지 않을 수 있기 때문이다.
- effect로는 흐림효과나 생동감효과를 줄 수 있는데 각각
UIBlurEffect
,UIVibrancyEffect
로 만들 수 있다.
import UIKit
class SceneDelegate: UIResponder, UIWindowSceneDelegate {
var window: UIWindow?
// TODO: 1. UIVisualEffectView 생성
var blurView: UIVisualEffectView?
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
guard let _ = (scene as? UIWindowScene) else { return }
}
func sceneDidBecomeActive(_ scene: UIScene) {
// TODO: 3. 앱이 다시 활성화 상태가 되면 blurView를 superview (window)에서 제거한다
if let blurView = blurView {
blurView.removeFromSuperview()
}
}
func sceneWillResignActive(_ scene: UIScene) {
// TODO: 2. will resign active 상태가 호출되면 blurView를 window 크기로 잡아주고 window에 추가한다
guard let window = window else {
return
}
let effect = UIBlurEffect(style: .regular)
blurView = UIVisualEffectView(effect: effect)
blurView?.frame = window.frame
window.addSubview(blurView!)
}
}
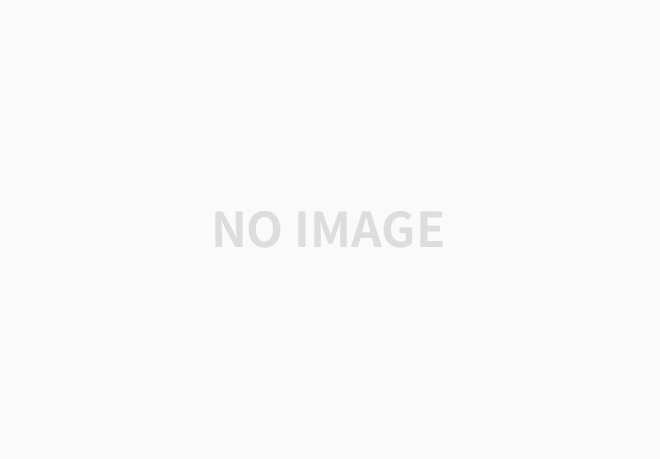
이상 App Switcher 로 진입 시 앱 화면을 가려주는 방법을 확인해봤다.
예제 프로젝트는 아래 깃헙에 :)
https://github.com/devssun/Wonder-Series-iOS/tree/master/AppSwitcherTest
반응형
'Programming > iOS' 카테고리의 다른 글
[iOS] MVC Pattern (0) | 2021.10.07 |
---|---|
[iOS/Swift] didFinishLaunchingWithOptions 알아보기, 푸시로 앱 실행할 때 처리하기 (0) | 2021.10.06 |
SwiftGen 라이브러리 간단 설명 & 사용법 (0) | 2021.07.12 |
[iOS 14] (내가 찾은) iOS 14 대응 할 거리들 (0) | 2020.12.22 |
[iOS 14] AppTrackingTransparency framework 이슈 처리하기 (0) | 2020.09.26 |